An analog phase shifter (or phaser) is one or more notch (band reject) filters modulated by a slow triangle or sine wave. It’s a fairly common effect, often used on guitar (Something About Us by Daft Punk) or keyboard (Are You All the Things by Bill Evans). The main character of the phase shifter is that of a quickly changing timbre, much like the sound of a Leslie rotating speaker. As it uses a moving notch filter, only a few of the harmonics are affected at any moment. Popular phasers include the MXR Phase 90, the Mu-Tron Bi-Phase, and the Electro Harmonix Small Stone.
Phase shifters are made by using a series of cascaded allpass filters, with the output of the last filter mixed with the input signal. The highest frequency should be shifted by a multiple of 2π and the lowest shifted by 0 in this group of allpass filters, so that the input and output signals are in phase at the top and bottom of the spectrum, and reinforce when mixed together.
Implementation
The first order allpass filter introduced earlier can be used for the cascaded filters in a phase shifter. As the first order allpass filter has a phase shift of π at nyquist, 2 of these filters are needed to obtain a phase shift of 2π.
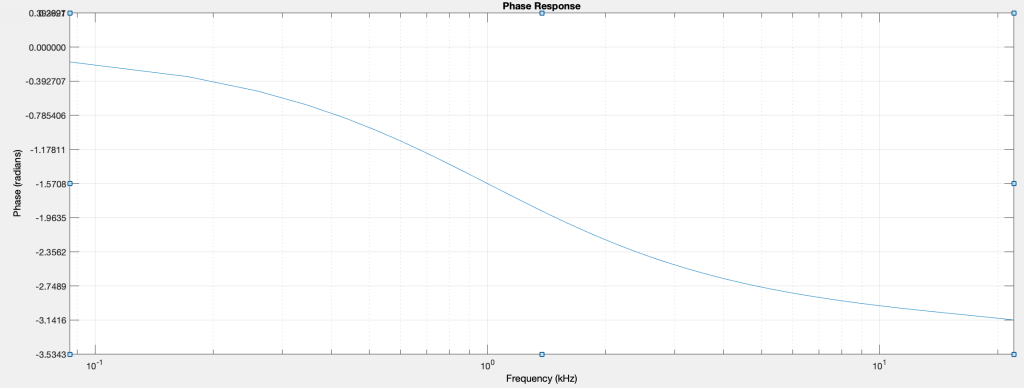
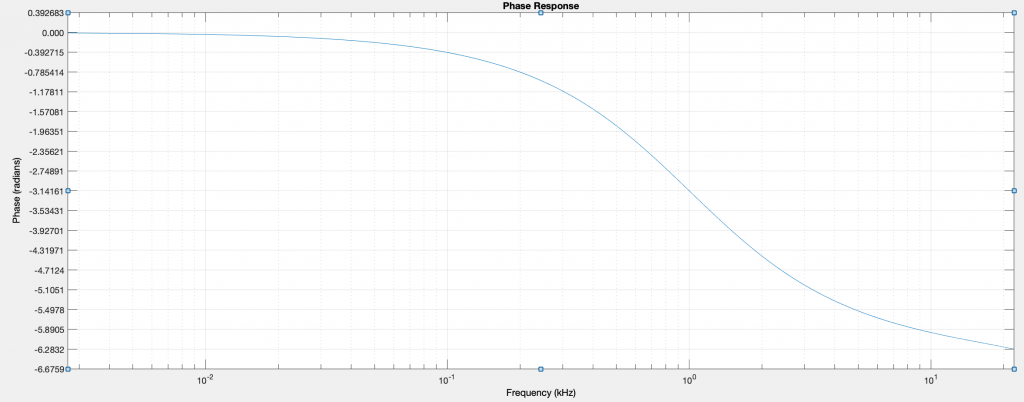
All of the filters in the cascade will have their frequency modulated by the same oscillator. This will move a -π (180) phase shift point up and down through the spectrum. When mixed with the input signal, the -π shift point will create the notch.
If more filters are cascaded, more notches will be created. Sounds at 0, -2π, -4π, -6π, etc. will be reinforced, and sounds at -π, -3π, -5π, etc. will be cancelled. The extra notches will create a deeper, more obviously effected sound, as more harmonics will be removed by the notches. Also important: the multiple notches are no longer harmonically related, and so the phase shifter will not act like a resonant comb filter.
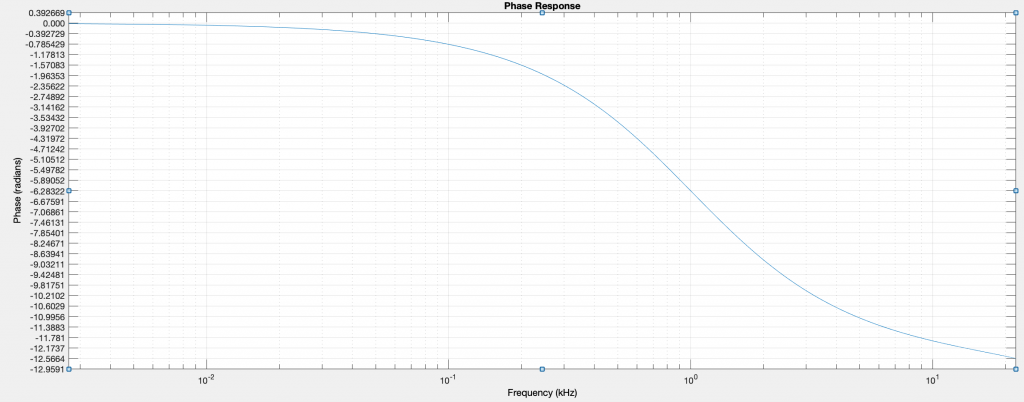
To put this together in C, a slow sine wave is needed. This will modulate the frequency of 4 cascaded first order allpass filters. The output of the last allpass filter will be mixed with the input signal.
// the samplerate in Hz float sampleRate = 44100; // the frequency of phase modulation - once every 2 seconds float frequency = 0.5; // initial phase float phase = 0.0; // x1 and y1 for each allpass stage double x1a, x1b, x1c, x1d; double y1a, y1b, y1c, y1d; void Phaser(float *input, float *output, float frequency, long samples) { long sample; float apfreq, tf, c; // calculate for each sample in a block for(sample = 0; sample < samples; sample++) { // get the phase increment for this sample phaseIncrement = frequency/sampleRate; // calculate the allpass frequency (200 to 5000) apfreq = (sin(phase * 6.283185307179586) + 1.0) * 2400.0 + 200; // calculate the allpass coefficient tf = tan(3.141592653589793 * apfreq/sampleRate); c = (tf - 1.0)/(tf + 1.0); // compute the 4 allpass filters, and save the previous input float x0a = *(input+i); y1a = (c*x0a) + x1a - (c * y1a); x1a = x0a; y1b = (c*y1a) + x1b - (c * y1b); x1b = y1a; y1c = (c*y1b) + x1c - (c * y1c); x1c = y1b; y1d = (c*y1c) + x1d - (c * y1d); x1d = y1c; // calculate the output for this sample *(output+sample) = *(input+sample) + y1d; // increment the phase phase = phase + phaseIncrement; if(phase >= 1.0) phase = phase - 1.0; } }