Decimation is a type of waveshaping that reduces the resolution of the input signal by eliminating the lower bits in the integer representation of the sample. For example, lets take a sample of value 0.5337 (within the typical range of 1.0 to -1.0) to 3 bit resolution. With 3 bits, we only have 8 values from -1.0 to 1.0: -1.0. -0.75, -0.5, -0.25, 0.0, 0.25, 0.5, 0.75. The value 0.5337 becomes 0.5 (as do all values between 0.5 and 0.75). This reduction in resolution will create noise which is not related to the amplitude of the signal. The less bits used, the great the decimation noise. If we consider 3-bit decimation a transfer function, it would look like this:
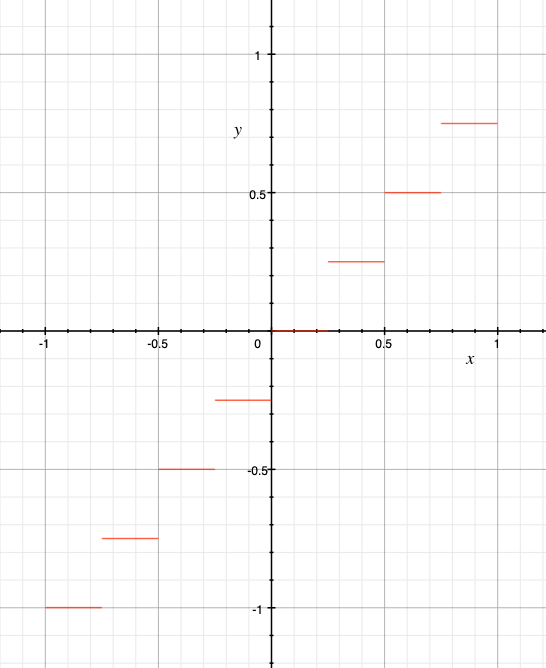
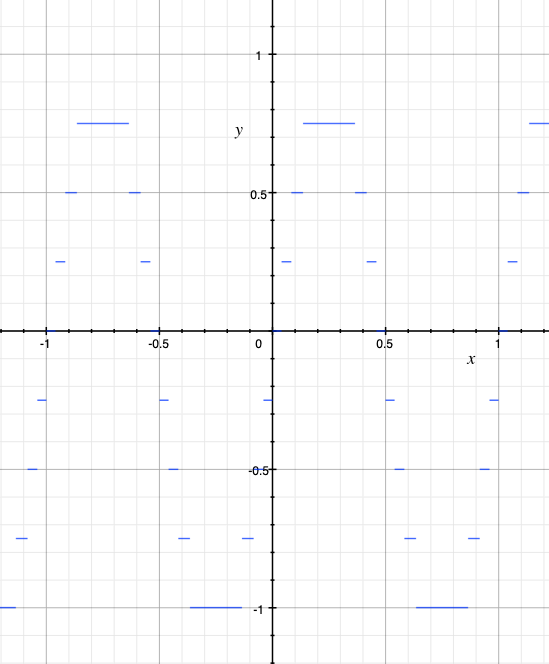
Using a transfer function like the above is one method of decimation. However, as with other types of waveshaping, it is more efficient to compute decimation directly, using the equation which would create the transfer function.
Implementation
The following equation could be used to decimate a floating point signal.
y[n] = \lfloor(x[n] * 2^{(bits - 1)})\rfloor/(bits-1)Below are two C implementations of decimation to 3 bits. First using multiplication and division, and the second using logical bit operations. The logical operations are probably much less CPU intensive.
// decimation to 3 bits using multiplication and division factor = pow(2.0, bits - 1.0); output = floor(input * factor)/factor; // decimation to 3 bits using logical operations // convert to 16-bit sample input16 = (int)(input * 32768); // zero out all but the top 3 bits using bitwise and // binary literals only allowed in gcc, in hex use 0xe000 output16 = input16 & 0b1110000000000000; // convert back to float using 1/32768 output = output16 * 0.000030517578125;
The first technique is more clear, and more flexible. It is also probably not that much more CPU intensive. Finally, the first technique could use a floating point value for bits, and this would allow continuous change from one bit depth to another.
When using decimation on recorded audio, you will notice that there is always distortion in the audio, and also that at low bit depths only the loudest sounds will be audible.